Python Code Performance Analysis
Discover how to analyze and optimize Python code performance with tools like cProfile, Py-Spy, and Timeit. Learn key optimization techniques for improving execution time and memory management.
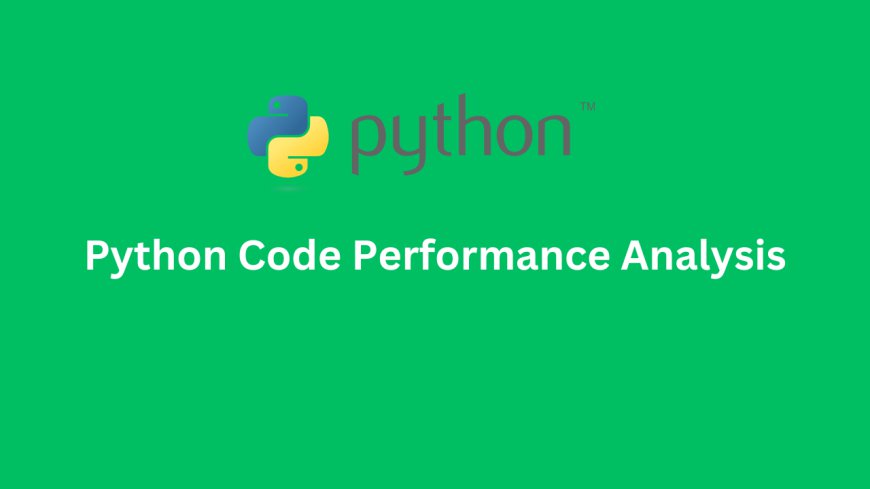
Introduction
Python is among the powerful, multi-purpose programming languages. However, one of the major criticisms leveled against Python is that it has a rather slow speed compared to other languages such as C or Java. This is the point where performance analysis in Python code plays an important role.
Whether you're developing a small script or working on a large application, performance analysis and optimization of your Python code will definitely yield great improvements in performance.
We will review, in this article, the different factors affecting Python performance, analysis tools, optimization techniques, and real-world examples. By the end of this, you shall have a comprehensive guide through which to understand how your code works, and how to improve the performance of your code written in Python.
Factors Affecting Python Performance
Characteristics of the Language
Python is an interpreted, dynamically typed language. This, in other words, means that code executes line by line. There can come performance bottlenecks, especially in computation-bound tasks.
Interpreter vs Compiler
Python is usually an interpreted language, meaning that it generally has not been executed by compiling it into machine code. This partly explains why Python execution tends to be slower compared to languages like C, whose code directly goes to compilation.
Assess and consolidate your Python scripts by utilizing online platforms like Python Online Compiler.
Profiling Tools for Performance Analysis in Python
Profiling Tool: cProfile, Py-Spy, and Line_profiler
Profiling tools can identify those parts of the code that involve the greatest consumption of resources. The most widely used are cProfile, a very complete one for global profiling; Py-Spy, which is very good with visuals; and Line_profiler, which works well on a line-by-line basis.
Timeit: Measuring Execution Time
Timeit is a standard Python library that allows the user to measure the execution time of small pieces of code. You will be able to test with it the performance under different conditions.
Code Optimization Techniques
Algorithmic Efficiency
Optimizing your code begins with using an efficient algorithm, like where an O(n^2) algorithm can be replaced by an O(n log n) one.
Data Structures
Generally speaking, selection of proper data structure considering the problem plays a pivotal role. Lists, sets, and dictionaries have various different time complexities for insertion, deletion, and lookup.
Memory Management
Python has automatic garbage collection; however, it can be important to optimize memory usage for performance-critical applications, which are bound by memory rather than CPU.
Profiling with cProfile
Installing and Running cProfile
The usage of cProfile is relatively straightforward, where you initiate your script execution with the -m cProfile option. For example:
-m cProfile my_script.py
Explanation of cProfile Output
The output of cProfile provides data in terms of number of calls and time spent inside a function and the number of calls. This could be quite useful for diagnostics on exactly where the performance bottlenecks are.
Visualization with Py-Spy
Py-Spy Capabilities
Py-Spy does performance profiling in real time by building flame graphs, a visualization that helps explain how many types of time have been consumed during code runtime.
How to Read Flame Graphs
Flame graphs identify the hottest points of your code, that is, the functions in which most of your execution time is consumed.
Line-by-Line Profiling with Line_profiler
Getting to Know Line_profiler
Line_profiler also provides information about the time each line of your code takes to execute, in order to highlight lines which are taking the longest time to execute and how you could go about optimizing it.
Line Profiling
Line_profiler does line-by-line profiling by using decorators that analyze a function's execution time and tells you exactly where in your code things are going slow.
Timing Execution with Timeit
Timeit Basics and Use Cases
The Timeit library can be used to measure execution time for small pieces of code. It's ideal for benchmarking small snippets and comparing different implementations.
Different Implementations
As an example, you might use Timeit to compare different looping, or data access patterns in Python to find which one is most efficient.
Memory Optimization Techniques
Python Memory Management
Python's memory consumption is best optimized by the optimization of data structures besides using tools such as memory_profiler for tracking memory consumption.
Using Generators for Efficiency
Generators allow for lazy evaluation, meaning values are generated on-demand and can provide memory efficiency if you're working with very large datasets.
Handling Very Large Datasets
When working with very large data sets, consider using libraries such as Pandas that have been optimized for memory and performance.
Algorithm Optimization
Understanding Algorithmic Complexity
Big-O notation denotes how algorithm complexity, with the increase in input size, grows. This is the best way to describe algorithmic complexity.
Performance Optimization Common Algorithms
Through performance optimization algorithms, such as Merge Sort or Quick Sort and optimizing look-up/ search operations, one can achieve immense gains.
Optimizing I/O Operations
Reading/Writing Files Efficiently
Based on the size of the files, using buffered I/O or reading/writing in chunks can give a tremendous performance boost.
Handling Network I/O
With network-bound tasks, asynchronous I/O can significantly improve performance, especially in web applications.
Multithreading and Multiprocessing
Multithreading in Python
Python's GIL, or Global Interpreter Lock, limits true parallelism in multithreading but still proves useful for I/O-bound tasks.
Advantages of Multiprocessing
For CPU-bound tasks, the multiprocessing module allows true parallel execution by instantiating multiple processes, thereby avoiding the GIL altogether.
Case Study: Real-World Example
A company was experiencing performance issues with the data processing pipeline in Python. By using cProfile profiling, they managed to optimize algorithms in memory consumption; thereby reducing the execution time by 50%.
Emerging Trends in Python Performance
New Performance Features in Recent Python Versions
Recent Python versions have added features to make function calls faster and handle garbage collection optimally for better performance.
Role of PyPy for Performance
Another implementation of the Python interpreter is PyPy. PyPy introduces just-in-time compilation, which significantly speeds up execution. Based on testing and measurements, that was where the greatest performance gains were to be found.
Conclusion
Performance analysis of Python code is an integral part of writing successful applications. You can easily do this using the tools cProfile, Py-Spy, and Timeit in addition to many other skills such as optimization of algorithms and managing memory that all will give a great performance boost to your Python code.
FAQs
What is Python code performance?
Performance in Python relates to the effectiveness of a Python script with respect to time and memory consumption.
How would I optimize the performance of my Python code?
You can optimize the performance of Python scripts through profiling tools, which aid in locating the bottlenecks; selection of algorithms with regard to efficiency; and utilization of resources with regard to memory efficiently.
What are the most used performance analyzers for Python?
The well-known ones are cProfile and Py-Spy, and Line_profiler for profiling, and Timeit for measuring execution time.