Robust Laravel Application Development - Lessons Learned
Discover key lessons and strategies for scaling Laravel Applications, focusing on optimizing performance & maintaining security.
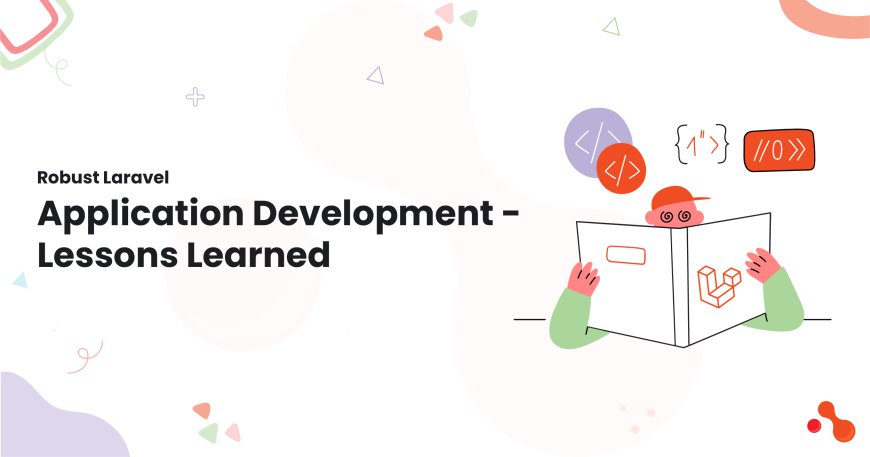
Introduction
Building a robust Laravel application that can scale, handle high traffic, and remain maintainable over time is not without its challenges. Over the years, developers and teams have encountered several common pitfalls and learned invaluable lessons while building and maintaining large-scale Laravel applications.
Developing robust applications with Laravel requires careful planning, attention to detail, and adherence to best practices. It is also essential to have an in-depth understanding of the intricacies of secure and scalable architecture. This would ideally mean trusting a software development outsourcing company like Acquaint Softtech.
This article provides more information on the lessons learned from building robust Laravel applications. It focuses on best practices, common mistakes, and solutions to improve application scalability, security, maintainability, and performance.
Laravel Application Development
Laravel has rapidly become one of the most popular PHP frameworks due to its expressive syntax, rich ecosystem, and developer-friendly features. With Laravel, developers can build modern web applications with ease, thanks to the abundance of built-in tools, comprehensive documentation, and support for modern PHP standards.
It is a powerful PHP framework that offers developers a structured and efficient way to build web applications. There are many reasons why Laravel is the ideal web framework. It has an elegant syntax, follows the MVC architecture, and has a special Blade templating engine.
Besides this, Laravel also eliminates the need to build an authentication system from scratch since it is in-built. The special object-relational mapping, Eloquent CRM helps simplify the database operations.
But that's not all. Not only does Laravel have good features to build a robust solution, but it also has the necessary tools and features to help you test it. Testing, debugging, and validation are highly simplified with Lararel. It allows the use of special test tools like PHPUnit and PEST.
Here are a few interesting statistics:
- 2706 of the Laravel powered websites are from the Web Development sector. (6sense)
- Over 303,718 Americans use Laravel, while this figure is 54,648 in the United Kingdom and 31,053 in Russia. (BuiltWith)
- As data collected in 2024, 7.9% of developers use the Laravel framework worldwide while 4.7% use Ruby on Rails, 3.2% use Symfony framework and 1.7% use Codeigniter.
- Node.js is one of the most popular with 40.8% using it; React comes close second at 39.5%
Lessons Learned
Understand the MVC Architecture Thoroughly : Lesson 1
Laravel adheres to the Model-View-Controller (MVC) architecture, which separates the application logic from the user interface. This separation is critical for building scalable and maintainable applications. One of the key lessons learned from working with Laravel is that a deep understanding of the MVC architecture is essential for building a robust application.
- Model: Represents the data layer and handles business logic.
- View: The user interface that the end-user interacts with.
- Controller: Mediates between the Model and the View, processing user requests and sending the appropriate response.
A common mistake is allowing business logic to seep into the controller or view. This breaks the MVC pattern, making the application harder to maintain and test. Adhering to strict separation of concerns ensures that each application part can be developed, tested, and maintained independently.
- Key Takeaway: Always ensure that business logic resides in models or services, controllers handle user requests, and views strictly present data without containing any application logic.
Use Eloquent ORM Effectively : Lesson 2
Laravel's built-in Object Relational Mapping (ORM) tool (Eloquent) provides a simple and elegant way to interact with the database. However, improper use of Eloquent can lead to performance issues, especially in large applications with complex database queries.
- Common Mistakes: N+1 Query Problem: The N+1 problem occurs when a query retrieves a list of items but then executes additional queries to fetch related items for each entry. This can lead to hundreds of unnecessary database queries. To avoid this, Laravel offers the with() method for eager loading relationships.
php code
// Bad
$posts = Post::all();
foreach ($posts as $post) {
echo $post->author->name;
}
// Good (Using eager loading)
$posts = Post::with('author')->get();
foreach ($posts as $post) {
echo $post->author->name;
}
Mass Assignment Vulnerabilities: Laravel's MassAssignment allows you to bulk insert or update data in the database. However, safeguarding against mass assignment can expose your application to vulnerabilities. Developers should always use $fillable or $guarded properties in their models to protect against mass assignment.
php code
// Protecting against mass assignment
protected $fillable = ['title', 'content', 'author_id'];
- Solutions: Always use eager loading to avoid the N+1 problem.
Define fillable attributes to protect against mass assignment.
Avoid complex queries in models. Instead, use Query Builder for more advanced database interactions that Eloquent struggles with.
- Key Takeaway: Use Eloquent for simple CRUD operations, but be mindful of performance concerns and security risks. When necessary, fall back on Laravel’s Query Builder for complex queries.
Service Layer for Business Logic : Lesson 3
As Laravel applications grow, keeping controllers slim and models focused on data becomes increasingly important. This is where the service layer comes in. Service classes allow developers to isolate business logic from controllers and models, making the application more modular and easier to test.
Example:
Instead of handling user registration logic in the controller, a service class can be created to handle it.
PHP code
// In the UserController
public function store(UserRequest $request)
{
$this->userService->registerUser($request->all());
}
// In UserService
public function registerUser(array $data)
{
$user = User::create($data);
// Other business logic related to registration
}
This pattern makes it easier to maintain, test, and scale the application as business logic becomes more complex.
- Key Takeaway: Introduce a service layer early on for business logic to improve code organization and maintainability.
Prioritize Security at Every Step : Lesson 4
Security is a fundamental aspect of building a robust Laravel application. One of the lessons learned from years of Laravel development is that security should not be an afterthought. Laravel provides many built-in security features, but it’s up to developers to use them correctly.
Key Security Practices:
Sanitize Input: Always sanitize user inputs to prevent XSS (Cross-Site Scripting) and SQL injection attacks. Laravel automatically escapes output in Blade templates, which helps protect against XSS. For SQL injection protection, always use Eloquent or Query Builder.
- Use CSRF Protection: Laravel automatically protects against Cross-Site Request Forgery (CSRF) by including a CSRF token in forms. Developers must ensure that CSRF protection is not disabled unintentionally.
- Authentication and Authorization: Laravel offers built-in authentication and authorization mechanisms through policies, gates, and middleware. Use them to control access to resources and ensure users have the necessary permissions.
- Password Hashing: Always store user passwords using Laravel’s Hash facade, which uses bcrypt by default. Avoid storing plain-text passwords in the database.
PHP
$user->password = Hash::make($request->password);
- Rate Limiting: To prevent brute force attacks, implement rate limiting using Laravel’s RateLimiter facade.
- Secure API Development: When building APIs, use OAuth2 or Laravel Sanctum for token-based authentication. Also, rate limiting for API endpoints should be implemented to prevent abuse.
- Key Takeaway: Leverage Laravel's built-in security features and be proactive in identifying potential vulnerabilities.
Lesson 5: Cache Strategically for Performance
Caching is critical for improving the performance of Laravel applications, especially as they scale. Laravel supports various caching mechanisms, including file, database, Redis, and Memcached.
Best Practices for Caching:
- Cache Expensive Queries: If your application frequently runs heavy queries, consider caching the results to reduce the load on the database.
PHP code
$users = Cache::remember('users', 60, function () {
return User::all();
});
- Route Caching: Laravel provides a route:cache Artisan command to cache all routes in your application. This can significantly improve the performance of larger applications.
Bash CODE
php artisan route:cache
- View Caching: Similarly, views can be cached to avoid unnecessary recompilation.
Bash CODE
php artisan view:cache
- Optimize Configuration Loading: Use config:cache to combine all configuration files into a single file, reducing the time it takes to load configurations during requests.
bash CODE
php artisan config:cache
- Key Takeaway: Use caching strategically to optimize performance and reduce server load, especially for database-heavy applications.
Optimize Database Interactions : Lesson 6
Efficient database interaction is critical for building scalable Laravel applications. Poorly optimized queries or over-reliance on ORM abstractions can lead to performance bottlenecks.
Best Practices for Database Optimization:
- Database Indexing: Ensure that frequently queried columns are indexed. Laravel’s migration system supports creating indexes with ease.
php code
Schema::table('users', function (Blueprint $table) {
$table->index('email');
});
- Avoid SELECT * Queries: Always specify the columns you need in a query. Fetching all columns unnecessarily increases the load on the database and memory usage.
php code
$users = User::select('id', 'name', 'email')->get();
- Use Pagination: For large datasets, always paginate results rather than fetching everything at once.
php code
$users = User::paginate(15);
- Database Query Profiling: Use Laravel’s built-in query log to identify slow queries. You can enable query logging with the following code:
php code
DB::enableQueryLog();
- Key Takeaway: Optimize database queries by using indexes, avoiding unnecessary data fetching, and employing efficient pagination techniques.
Test Your Application : Lesson 7
Testing is a crucial aspect of robust Laravel application development. Laravel comes with PHPUnit integration, making it easy to write unit tests, feature tests, and browser tests. However, many developers tend to skip or neglect testing due to time constraints, which often leads to bugs and regressions.
Testing Best Practices:
- Unit Tests: Unit tests focus on testing individual components or methods. They are fast and reliable but don’t cover the whole application flow.
php code
public function testUserCreation()
{
$user = User::factory()->create();
$this->assertDatabaseHas('users', ['email' => $user->email]);
}
- Feature Tests: Feature tests allow you to test the entire application flow, from requests to responses. They ensure that the application behaves as expected in real-world scenarios.
php code
public function testUserRegistration()
{
$response = $this->post('/register', [
'name' => 'John Doe',
'email' => 'john@example.com',
'password' => 'secret',
'password_confirmation' => 'secret',
]);
$response->assertStatus(302);
$this->assertDatabaseHas('users', ['email' => 'john@example.com']);
}
- Continuous Integration: Integrate automated tests with a continuous integration (CI) tool such as GitHub Actions or Travis CI. This ensures that your tests are automatically run whenever new code is pushed, preventing broken code from being deployed.
- Key Takeaway: Always write tests for critical application components and workflows to ensure stability and reliability.
Prioritize Code Maintainability and Readability : Lesson 8
Laravel is known for its elegant syntax and developer-friendly structure, but as the application grows, maintaining clean and readable code becomes challenging.
- Best Practices for Maintainability:
- Follow PSR Standards: Laravel encourages following the PHP-FIG’s PSR standards, such as PSR-2 for coding style and PSR-4 for autoloading. Following these standards ensures that your codebase remains consistent and easy to navigate.
- Use Named Routes: Using named routes instead of hard-coded URLs makes it easier to manage changes in the URL structure.
php code
Route::get('/user/profile', 'UserProfileController@show')->name('profile');
- Refactor Regularly: As the application evolves, refactor code regularly to avoid technical debt. Use Laravel’s php artisan make:command to create custom Artisan commands that automate repetitive tasks.
- Comment and Document: Clear comments and documentation help other developers (or even yourself in the future) understand the purpose of the code. Use Laravel’s DocBlocks to document classes, methods, and variables.
- Key Takeaway: Invest in code quality by following coding standards, refactoring regularly, and documenting thoroughly.
Leverage Laravel’s Ecosystem : Lesson 9
Laravel has an extensive ecosystem that can simplify development and add powerful features to your application. Leveraging this ecosystem can save Laravel application development time and reduce complexity.
Key Laravel Tools and Packages:
- Laravel Horizon: Provides a dashboard and tools to monitor queues and jobs in real-time.
- Laravel Echo: Integrates WebSockets and real-time broadcasting capabilities into Laravel applications.
- Laravel Telescope: A debugging assistant that allows you to monitor requests, queries, exceptions, logs, and more.
- Laravel Sanctum: API authentication using tokens is especially useful for single-page applications (SPAs).
- Laravel Vapor: A serverless deployment platform for Laravel that enables you to deploy Laravel applications on AWS Lambda without managing infrastructure.
- Key Takeaway: Use Laravel’s ecosystem of tools and packages to extend your application’s capabilities and streamline development.
Prepare for Scalability : Lesson 10
As your Laravel application grows, you need to ensure that it can scale to handle increased traffic and data.
Key Scalability Considerations:
- Horizontally Scale Your Database: As your user base grows, consider using database replication to scale horizontally. Laravel supports multiple database connections and read-write splitting.
- Use Job Queues: Offload time-consuming tasks (such as sending emails or processing files) to queues. Laravel’s queue system supports drivers like Redis, Beanstalkd, and Amazon SQS.
- Optimize Load Balancing: For high-traffic applications, consider using a load balancer to distribute incoming traffic across multiple application servers.
- Containerization: Use Docker to containerize your Laravel application. Containerization ensures that your application can run consistently across various environments and can be scaled easily using orchestration tools like Kubernetes.
- Key Takeaway: Plan for scalability from the start by using job queues, load balancing, and database replication.
Use Configuration and Environment Management Properly : Lesson 11
Lesson: Mismanaged environment configurations can lead to security and stability issues.
- Practice: Store sensitive configurations like database credentials, API keys, and service configurations in .env files. Utilize Laravel’s configuration caching (config:cache) for production environments.
- Tip: Regularly audit .env files and restrict sensitive data from being logged or committed to version control.
Some of the other lessons learned from developing a robust Laravel solution include that it has Artisan. This allows one to use a command line tool, Artisan, that can automate repetitive tasks. This serves the purpose of accelerating the development process. It is possible to simplify the process of managing the packages with the help of 'Composer'. API development is a breeze with Laravel since it has in-built support for RESTful APIs.
Benefits Of A Robust Laravel Solution
A robust Laravel solution offers a multitude of benefits for businesses and developers, ranging from enhanced security and scalability to faster development and easier maintenance.
Hire remote developers from Acquaint Softtech to build a top-notch solution. We have over 10 years of experience developing cutting-edge solutions.
Here's a detailed look at the key benefits of using a robust Laravel solution:
- Scalability and Performance Optimization
- Enhanced Security Features
- Faster Time to Market
- Modular and Maintainable Codebase
- Seamless API Integration
- Efficient Database Management
- Simplified User Authentication and Authorization
- Robust Testing Capabilities
- Efficient Task Scheduling and Background Processing
- Vibrant Community and Ecosystem
- Advanced Caching Mechanisms
- Support for Multilingual and Multi-tenant Applications
- Built-in Tools for SEO and Content Management
Conclusion
Building a robust Laravel application requires a deep understanding of best practices, design patterns, and the ability to anticipate challenges. By learning from the lessons shared above, developers can avoid common pitfalls and ensure that their applications are secure, performant, maintainable, and scalable. Following these guidelines not only enhances the quality of the code but also helps deliver applications that stand the test of time
Analyzing case studies of successful Laravel applications can provide a roadmap for best practices and strategies. At the same time, these lessons learned from developing a robust, scalable, and secure Laravel application are also of great value.