Pointers in C Programming with Examples
Pointers are a fundamental concept in C programming that provides powerful features for managing memory and manipulating data efficiently.
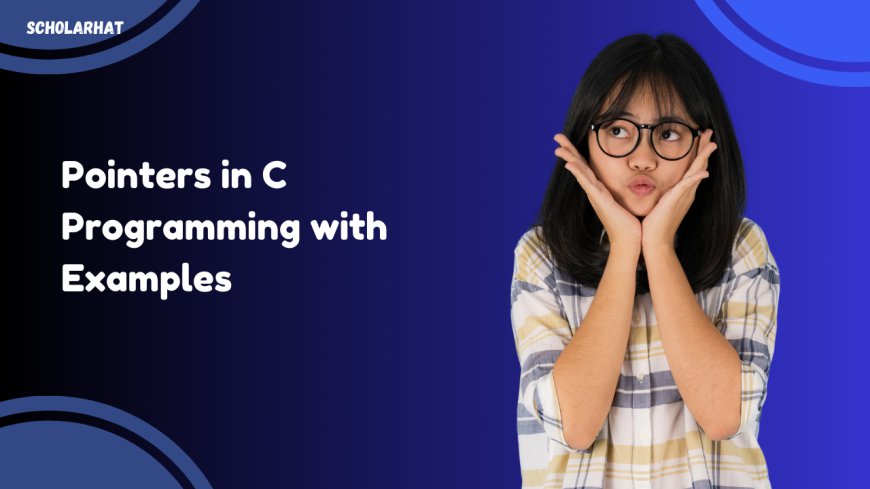
Pointers are a fundamental concept in C programming that provides powerful features for managing memory and manipulating data efficiently. Understanding pointers is crucial for both beginners and experienced programmers to write optimized and effective code.
This article will delve into the basics of pointers in C, provide examples, and compare them with similar concepts in C++ such as C++ strings and pointers in cpp.
What are Pointers?
A pointer in C++ is a variable that stores the memory address of another variable. Instead of holding a value directly, a pointer points to the location where the value is stored.
Key Features of Pointers
-
Direct Memory Access: Pointers allow direct access to memory, which can be useful for low-level programming.
-
Dynamic Memory Allocation: Pointers are essential for dynamic memory allocation using functions like malloc and free.
-
Efficient Array Handling: Pointers enable efficient manipulation and passing of arrays and strings.
Declaring and Initializing Pointers
To declare a pointer, you use the * operator. Here’s a basic example:
int *ptr; int var = 10; ptr = &var;
Accessing Values Using Pointers
You can access the value stored at the memory address pointed to by a pointer using the dereference operator (*):
#include <stdio.h>
int main() {
int var = 10;
int *ptr = &var;
printf("Value of var: %d\n", var); // Output: 10
printf("Value using pointer: %d\n", *ptr); // Output: 10
return 0;
}
Pointers and Arrays
Pointers and arrays are closely related. An array name acts as a pointer to its first element. Here’s an example:
#include <stdio.h>
int main() { int arr[3] = {10, 20, 30}; int *ptr = arr;
for (int i = 0; i < 3; i++) {
printf("Value at arr[%d]: %d\n", i, *(ptr + i));
}
return 0;
}
Pointer Arithmetic
Pointer arithmetic allows you to traverse arrays and manipulate memory addresses. For instance, incrementing a pointer moves it to the next memory location of its type:
#include <stdio.h>
int main() { int arr[3] = {10, 20, 30}; int *ptr = arr;
printf("Address of arr[0]: %p\n", ptr);
printf("Address of arr[1]: %p\n", ptr + 1);
printf("Address of arr[2]: %p\n", ptr + 2);
return 0;
}
Comparison with C++ Strings and Pointers
In C++, the C++ string class provides a more abstract way of handling strings compared to C strings (character arrays). However, pointers are still widely used in C++ for various purposes, similar to C.
Example: Pointers in C++
#include <iostream>
int main() { int var = 10; int *ptr = &var;
std::cout << "Value of var: " << var << std::endl;
std::cout << "Value using pointer: " << *ptr << std::endl;
return 0;
}
Example: C++ String with Pointers
#include <iostream> #include <string>
int main() { std::string str = "Hello, World!"; std::string *ptr = &str;
std::cout << "Value of str: " << str << std::endl;
std::cout << "Value using pointer: " << *ptr << std::endl;
return 0;
}
Table: Key Differences between C and C++ Pointers
Feature |
C Pointers |
C++ Pointers |
Syntax |
int *ptr; |
int *ptr; |
String Handling |
Character arrays (char arr[] = "Hello";) |
std::string class |
Memory Management |
Manual (malloc, free) |
Manual (new, delete) |
Type Safety |
Less type-safe |
More type-safe with features like smart pointers |
Standard Library Support |
Limited |
Extensive (STL, smart pointers, etc.) |
Practical Examples
Example 1: Swapping Values Using Pointers
#include <stdio.h>
void swap(int *a, int *b) { int temp = *a; *a = *b; *b = temp; }
int main() { int x = 10, y = 20; swap(&x, &y);
printf("After swap: x = %d, y = %d\n", x, y); // Output: x = 20, y = 10
return 0;
}
Example 2: Dynamic Memory Allocation
#include <stdio.h> #include <stdlib.h>
int main() { int *ptr; int n, i;
printf("Enter number of elements: ");
scanf("%d", &n);
ptr = (int*)malloc(n * sizeof(int));
if (ptr == NULL) {
printf("Memory not allocated.\n");
exit(0);
}
printf("Memory successfully allocated using malloc.\n");
for (i = 0; i < n; ++i) {
ptr[i] = i + 1;
}
printf("The elements of the array are: ");
for (i = 0; i < n; ++i) {
printf("%d ", ptr[i]);
}
free(ptr);
return 0;
}
Conclusion
Pointers in C are a powerful tool that offers flexibility and control over memory management and data manipulation. While they require careful handling to avoid issues like memory leaks and segmentation faults, mastering pointers is essential for any C programmer. Additionally, understanding the differences and similarities between pointers in C++ and C++ string can provide a broader perspective on their usage across different programming languages.