What Is Exception Handling in C++? Explain With Example
Exception handling in C++ is a powerful feature that allows developers to manage errors and exceptional situations in a structured and controlled manner.
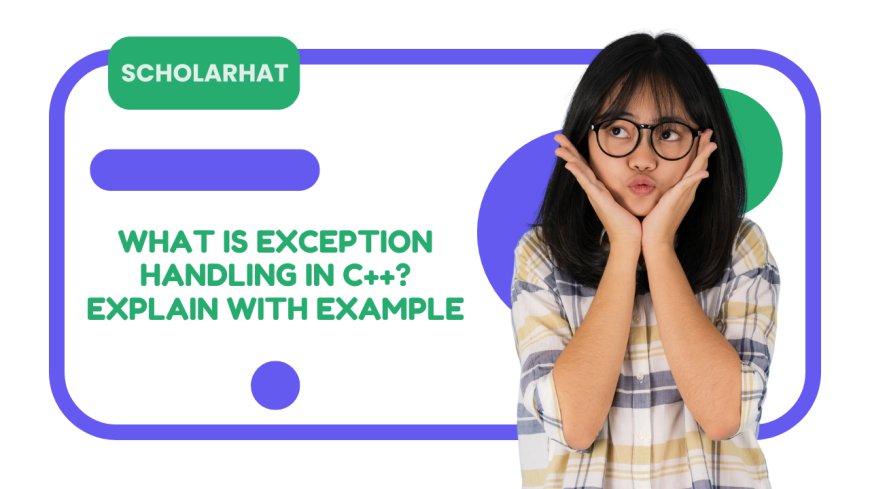
Exception handling in C++ is a powerful feature that allows developers to manage errors and exceptional situations in a structured and controlled manner. It helps in writing robust and error-free code by separating error-handling code from the regular logic.
In this article, we will explore the concept of exception handling in C++ with a detailed example and practical insights.
What Is Exception Handling?
Exception handling is a mechanism to handle runtime errors, providing a way to react to exceptional circumstances (like runtime errors) in a program. The main idea is to ensure that the flow of the program can be maintained even when unexpected situations occur.
Key Concepts of Exception Handling in C++
-
Try Block: Contains the code that may throw an exception.
-
Catch Block: Catches and handles the exception thrown by the try block.
-
Throw Statement: Used to throw an exception.
Why Use Exception Handling?
Using exception handling in C++ offers several benefits:
-
Improves Code Readability: Separates error-handling code from regular code.
-
Enhances Maintainability: Easier to manage and update error-handling routines.
-
Promotes Robustness: Ensures that resources are properly released and programs can handle unexpected situations gracefully.
Syntax of Exception Handling in C++
The basic syntax involves the try, catch, and throw keywords. Here is a simplified structure:
-
Try Block: Code that may cause an exception is placed inside a try block.
-
Catch Block: Code to handle the exception is placed inside a catch block.
-
Throw Statement: Used within the try block to throw an exception when an error occurs.
Example
Imagine you have a function that divides two numbers. If the denominator is zero, it should throw an exception:
try {
// Code that may throw an exception
int result = divide(num1, num2);
} catch (const char* msg) {
// Code to handle the exception
std::cerr << msg << std::endl;
}
Detailed Example
Let's consider a more detailed example where we handle division by zero:
Step-by-Step Explanation
-
Define a Function: Create a function to perform division.
-
Throw Exception: If the denominator is zero, throw an exception.
-
Try Block: Call the function within a try block.
-
Catch Block: Catch and handle the exception.
Function Definition
Create a function divide that throws an exception if the denominator is zero.
Try Block
Use the try block to call the divide function and handle any exceptions that may be thrown.
Catch Block
Use the catch block to catch the exception and display an appropriate message.
Example Code
The example code can be structured as follows:
int divide(int a, int b) {
if (b == 0) {
throw "Division by zero error";
}
return a / b;
}
int main() {
try {
int result = divide(10, 0);
} catch (const char* msg) {
std::cerr << msg << std::endl;
}
return 0;
}
Table: Exception Handling Keywords
Keyword |
Description |
try |
Block of code to monitor for exceptions |
catch |
Block of code to handle the exception |
throw |
Keyword used to throw an exception |
Benefits of Using Exception Handling
-
Separates Error Handling Code: Makes the code cleaner and easier to read.
-
Centralized Error Handling: One catch block can handle multiple types of errors.
-
Resource Management: Ensures resources like memory and file handles are properly managed.
Conclusion
Exception handling in C++ is an essential feature for managing errors and ensuring that programs can handle unexpected situations gracefully. By using try, catch, and throw statements, developers can write more robust and maintainable code. Understanding exception handling helps in writing programs that are resilient and can recover from errors effectively. Whether you are dealing with simple errors or complex error-handling scenarios, mastering exception handling in C++ is a valuable skill for any programmer.