What is the difference between a private and protected variable in Java?
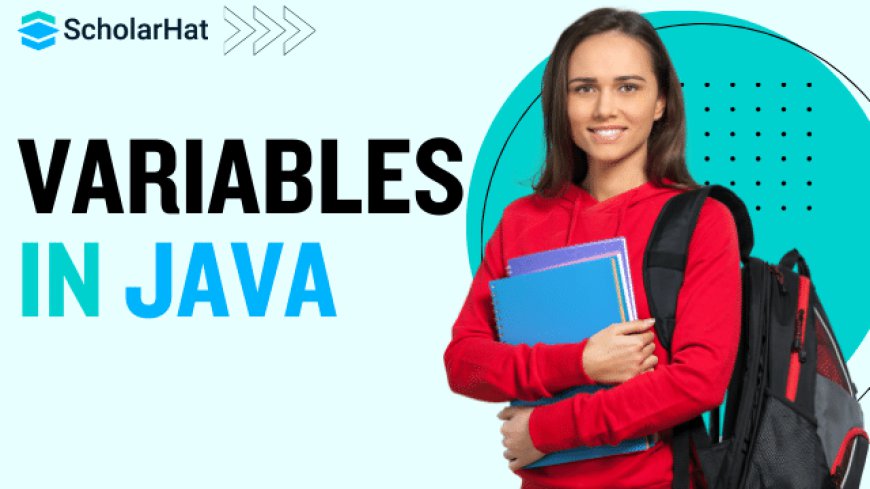
How can I call a class from another class in Java?
Unlockinag the Power of Inter-Class Communication: How to Call a Class from Another Class in Java
In the world of Java programming, understanding how to effectively communicate between classes is a fundamental skill that can greatly enhance your ability to create robust and modular applications. The concept of calling a class in Java from another class is essential for building complex software systems and implementing object-oriented design principles. This comprehensive guide will explore various methods and best practices for achieving seamless inter-class communication in Java, helping you elevate your programming skills and create more efficient, maintainable code.
Understanding the Basics of Java Classes
Before diving into the intricacies of calling one class from another, it's crucial to have a solid grasp of what classes are and how they function in Java.
What is a Class in Java?
A class in Java is a blueprint or template for creating objects. It encapsulates data for the object and methods to manipulate that data. Classes are the fundamental building blocks of object-oriented programming in Java, allowing developers to create modular, reusable code.
Components of a Java Class
-
Fields: Variables that hold the state of an object.
-
Methods: Functions that define the behavior of objects.
-
Constructors: Special methods used to initialize objects.
-
Nested Classes: Classes defined within another class.
The Importance of Inter-Class Communication
In real-world applications, it's rare for a single class to contain all the functionality needed for a program. Most often, you'll need to create multiple classes that work together to achieve the desired outcome. This is where the ability to call one class from another becomes crucial.
Benefits of Effective Inter-Class Communication
-
Modularity: Allows you to break down complex systems into manageable components.
-
Reusability: Enables the use of existing classes in multiple parts of your application.
-
Maintainability: Makes it easier to update and modify specific parts of your code without affecting the entire system.
-
Scalability: Facilitates the growth of your application by allowing for the easy addition of new features.
Methods for Calling a Class from Another Class
Now that we understand the importance of inter-class communication, let's explore the various methods you can use to call a class from another class in Java.
1. Creating an Instance of the Class
The most straightforward method of calling a class from another class is by creating an instance of the desired class and then calling its methods or accessing its fields.
java
Copy
public class MainClass {
public static void main(String[] args) {
SecondaryClass secondaryObj = new SecondaryClass();
secondaryObj.displayMessage();
}
}
class SecondaryClass {
public void displayMessage() {
System.out.println("Hello from SecondaryClass!");
}
}
In this example, we create an instance of SecondaryClass within MainClass and then call its displayMessage() method.
2. Using Static Methods
If the method you want to call doesn't require an instance of the class (i.e., it's a static method), you can call it directly using the class name, without creating an object.
java
Copy
public class MainClass {
public static void main(String[] args) {
SecondaryClass.staticDisplayMessage();
}
}
class SecondaryClass {
public static void staticDisplayMessage() {
System.out.println("Hello from a static method in SecondaryClass!");
}
}
Here, we call the static method staticDisplayMessage() directly on the SecondaryClass without creating an instance.
3. Inheritance
Inheritance allows a class to inherit properties and methods from another class. This is a powerful way to reuse code and establish relationships between classes.
java
Copy
public class ParentClass {
public void parentMethod() {
System.out.println("This is a method from the parent class.");
}
}
public class ChildClass extends ParentClass {
public void childMethod() {
System.out.println("This is a method from the child class.");
parentMethod(); // Calling the parent class method
}
}
In this example, ChildClass inherits from ParentClass and can call the parentMethod() directly.
4. Interfaces
Interfaces provide a way to achieve abstraction and define a contract that classes must follow. They can be used to establish relationships between classes that don't share a direct inheritance hierarchy.
java
Copy
public interface Drawable {
void draw();
}
public class Circle implements Drawable {
public void draw() {
System.out.println("Drawing a circle");
}
}
public class DrawingApp {
public void drawShape(Drawable shape) {
shape.draw();
}
}
Here, the DrawingApp class can work with any class that implements the Drawable interface, allowing for flexible inter-class communication.
Advanced Techniques for Inter-Class Communication
As you become more proficient in Java programming, you'll encounter more sophisticated ways of managing inter-class relationships and communication. Let's explore some advanced techniques that can enhance your code's flexibility and maintainability.
1. Dependency Injection
Dependency Injection (DI) is a design pattern that allows you to decouple classes by injecting dependencies from the outside, rather than having a class create its own dependencies.
java
Copy
public class EmailService {
public void sendEmail(String message) {
System.out.println("Sending email: " + message);
}
}
public class UserService {
private EmailService emailService;
public UserService(EmailService emailService) {
this.emailService = emailService;
}
public void registerUser(String username) {
// User registration logic
emailService.sendEmail("Welcome, " + username + "!");
}
}
public class Main {
public static void main(String[] args) {
EmailService emailService = new EmailService();
UserService userService = new UserService(emailService);
userService.registerUser("JohnDoe");
}
}
In this example, the EmailService is injected into the UserService, making the code more modular and easier to test.
2. Factory Pattern
The Factory Pattern is a creational pattern that provides an interface for creating objects in a superclass, allowing subclasses to decide which class to instantiate.
java
Copy
public interface Animal {
void makeSound();
}
public class Dog implements Animal {
public void makeSound() {
System.out.println("Woof!");
}
}
public class Cat implements Animal {
public void makeSound() {
System.out.println("Meow!");
}
}
public class AnimalFactory {
public Animal createAnimal(String animalType) {
if (animalType.equalsIgnoreCase("dog")) {
return new Dog();
} else if (animalType.equalsIgnoreCase("cat")) {
return new Cat();
}
return null;
}
}
public class Main {
public static void main(String[] args) {
AnimalFactory factory = new AnimalFactory();
Animal animal = factory.createAnimal("dog");
animal.makeSound();
}
}
This pattern allows for more flexible object creation and can simplify inter-class communication in complex systems.
3. Observer Pattern
The Observer Pattern is a behavioral design pattern that lets you define a subscription mechanism to notify multiple objects about any events that happen to the object they're observing.
java
Copy
import java.util.ArrayList;
import java.util.List;
interface Observer {
void update(String message);
}
class Subject {
private List<Observer> observers = new ArrayList<>();
private String state;
public void attach(Observer observer) {
observers.add(observer);
}
public void notifyAllObservers() {
for (Observer observer : observers) {
observer.update(state);
}
}
public void setState(String state) {
this.state = state;
notifyAllObservers();
}
}
class ConcreteObserver implements Observer {
private String name;
public ConcreteObserver(String name) {
this.name = name;
}
@Override
public void update(String message) {
System.out.println(name + " received message: " + message);
}
}
public class Main {
public static void main(String[] args) {
Subject subject = new Subject();
ConcreteObserver observer1 = new ConcreteObserver("Observer 1");
ConcreteObserver observer2 = new ConcreteObserver("Observer 2");
subject.attach(observer1);
subject.attach(observer2);
subject.setState("New State");
}
}
This pattern is particularly useful for implementing distributed event handling systems.
Best Practices for Inter-Class Communication
To ensure that your code remains clean, maintainable, and efficient, consider the following best practices when implementing inter-class communication:
1. Follow the Single Responsibility Principle
Each class should have a single, well-defined responsibility. This makes it easier to understand, modify, and reuse classes.
2. Use Interfaces for Abstraction
Interfaces allow you to define contracts between classes without tying them to specific implementations, promoting loose coupling.
3. Favor Composition Over Inheritance
While inheritance is powerful, it can sometimes lead to tight coupling between classes. Composition often provides more flexibility and easier maintenance.
4. Implement Proper Encapsulation
Use access modifiers (public, private, protected) judiciously to control access to class members and methods.
5. Utilize Design Patterns
Familiarize yourself with common design patterns and apply them where appropriate to solve recurring design problems.
6. Write Clean and Readable Code
Use meaningful names for classes, methods, and variables. Follow consistent coding standards and provide clear documentation.
7. Consider Thread Safety
If your application is multi-threaded, ensure that inter-class communication is thread-safe to avoid concurrency issues.
Common Pitfalls and How to Avoid Them
When implementing inter-class communication, be aware of these common pitfalls:
1. Circular Dependencies
Avoid situations where two or more classes depend on each other, creating a circular dependency. This can lead to tight coupling and make the code difficult to maintain.
Solution: Use interfaces, dependency injection, or restructure your code to break the circular dependency.
2. Excessive Coupling
Tightly coupled classes are difficult to modify and reuse. They often lead to a "ripple effect" where changes in one class necessitate changes in many others.
Solution: Strive for loose coupling by using interfaces, dependency injection, and following the SOLID principles.
3. God Objects
Avoid creating classes that try to do too much or know too much about other parts of the system.
Solution: Break down large classes into smaller, more focused classes with clear responsibilities.
4. Ignoring Access Modifiers
Improper use of access modifiers can lead to unintended access to class members, violating encapsulation.
Solution: Use the most restrictive access modifier possible for each class member and method.
5. Overusing Static Methods
While static methods have their place, overusing them can lead to procedural-style programming and make code harder to test and maintain.
Solution: Use instance methods when possible, and reserve static methods for utility functions or factory methods.
The Future of Inter-Class Communication in Java
As Java continues to evolve, we can expect to see new features and best practices emerge for inter-class communication. Here are some trends to watch:
1. Enhanced Modularity with Project Jigsaw
Project Jigsaw, introduced in Java 9, brings a new level of modularity to Java applications. This can significantly impact how classes interact across module boundaries.
2. Increased Use of Functional Programming Concepts
With the introduction of lambda expressions and the Stream API, Java is incorporating more functional programming concepts. This can lead to new patterns for inter-class communication.
3. Reactive Programming
The adoption of reactive programming models, such as Project Reactor or RxJava, is changing how classes communicate, especially in asynchronous and event-driven systems.
4. Improved Type Inference
Enhancements to Java's type inference capabilities may lead to more concise and readable code when working with generics and lambda expressions.
Conclusion: Mastering Inter-Class Communication for Robust Java Applications
Understanding how to effectively call a class from another class in Java is a crucial skill for any Java developer. It forms the foundation for building complex, modular, and maintainable applications. By mastering the various methods of inter-class communication - from simple object instantiation to advanced design patterns - you'll be well-equipped to tackle a wide range of programming challenges.
Remember that effective inter-class communication goes beyond just knowing the syntax. It requires a deep understanding of object-oriented principles, design patterns, and best practices. As you continue to develop your skills, focus on writing clean, modular code that adheres to the SOLID principles and promotes loose coupling between classes.
Keep in mind that the field of software development is constantly evolving. Stay curious, keep learning, and be open to new approaches and technologies. By doing so, you'll not only improve your ability to call classes from other classes but also become a more well-rounded and effective Java developer.
Whether you're building small applications or large-scale enterprise systems, the principles and techniques discussed in this guide will serve you well. Apply them thoughtfully, always considering the specific needs and constraints of your project. With practice and experience, you'll develop an intuitive sense for when and how to implement different inter-class communication strategies, allowing you to create robust, efficient, and maintainable Java applications.