The Key Strategies for Angular Change Detection
Discover essential strategies for mastering Angular change detection to enhance app performance. Learn about different detection strategies, best practices, and advanced techniques for building efficient Angular applications.
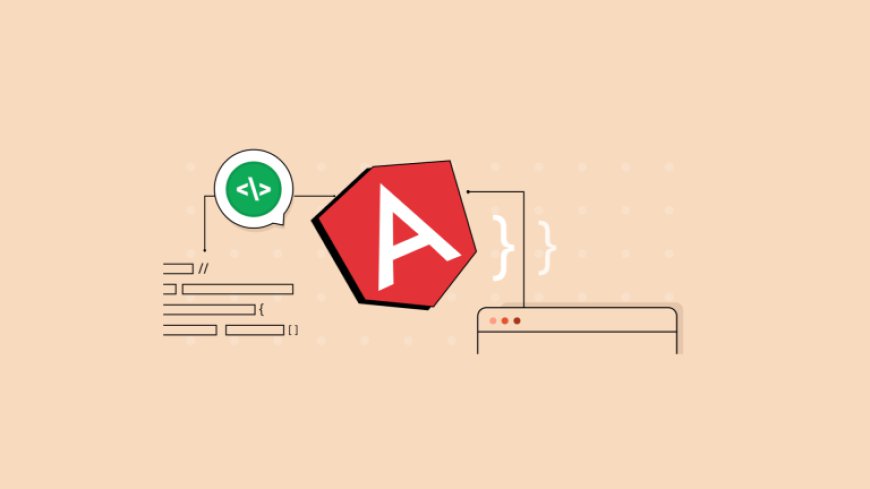
If you’re looking to optimize performance in your Angular applications, mastering change detection is essential. Angular's change detection mechanism enables applications to detect and respond to changes within the app's state, delivering a seamless user experience. Whether you're building a small app or a large-scale enterprise project, understanding and using the right change detection strategies can significantly improve efficiency. This guide will explore the ins and outs of Angular change detection strategies to help you make informed decisions.
What is Change Detection?
Change detection is a core concept in Angular that allows the framework to update the DOM (Document Object Model) when the state of the app changes. Essentially, it enables Angular to know when and where changes occur so that the UI can reflect them accurately. When change detection is triggered, Angular will update only the relevant components, which makes applications more efficient.
Understanding how change detection works is crucial if you want to hire AngularJS developers who can create high-performing applications, as it plays a significant role in how fast your app responds to user interactions.
How Change Detection Works in Angular
Angular uses a change detection mechanism based on a zone library, which helps it track changes across the application. Here's a simplified view of how it works:
- Angular monitors changes in variables, services, and DOM events.
- Whenever a change is detected, Angular performs a check on the component tree to update any affected views.
- By default, Angular’s change detection runs automatically, triggered by asynchronous events like HTTP requests, setTimeout, or user interactions.
Angular's default change detection strategy checks all components from top to bottom, which can sometimes be resource-intensive, especially in larger apps. Developers have the option to fine-tune this process by implementing different change detection strategies to improve efficiency.
Angular Change Detection Strategies
Angular offers two primary strategies for change detection: Default and OnPush. Each strategy has its advantages and trade-offs, so it’s crucial to understand when to use each one.
1. Default Strategy
In the default change detection strategy, Angular checks every component in the component tree for changes after every asynchronous event. This means that if you make an HTTP request or click a button, Angular will run change detection on all components, even those that haven’t been modified.
The advantage of this strategy is simplicity, as developers don’t need to configure anything manually. However, for applications with a deep component tree or many asynchronous operations, this strategy can lead to performance issues, as Angular may end up rechecking unchanged components repeatedly.
2. OnPush Strategy
The OnPush strategy allows Angular to skip certain components in the change detection cycle if their inputs haven’t changed. Components using the OnPush strategy will only be checked if:
- An @Input property changes
- An event inside the component occurs
- The component is manually marked for checking using Angular’s
markForCheck()
function
The OnPush strategy is ideal for performance-oriented applications, as it reduces the number of checks Angular performs. It’s especially useful for components that are primarily dependent on external inputs and don’t need to re-render frequently.
To implement OnPush, developers need to change the changeDetection
property in the component decorator:
@Component({
changeDetection: ChangeDetectionStrategy.OnPush,
...
})
export class MyComponent {}
If you hire AngularJS developers, ensure they are familiar with the OnPush strategy to help build efficient, scalable applications.
Implementing Angular Change Detection Strategies
When implementing change detection strategies in your application, it’s important to consider the complexity of your app’s component tree and the data flow between components. Here are some practical steps to get started:
Using ChangeDetectionRef for Precise Control
ChangeDetectorRef
is an Angular service that provides control over change detection at a granular level. Here are some commonly used methods:
- detectChanges(): Triggers change detection for the component.
- markForCheck(): Marks the component and its ancestors for checking in the next change detection cycle.
- detach(): Detaches the component from the change detection cycle, preventing it from being checked automatically.
Smart Use of Observables
When working with reactive data, Observables can play an essential role in optimizing change detection. Using async
pipes with Observables can minimize manual handling of subscriptions and ensure Angular handles change detection efficiently.
Avoiding Unnecessary Change Detection with detach()
In some cases, detaching a component from Angular’s change detection tree using detach()
can improve performance. This approach is particularly useful in scenarios where the component is not expected to change frequently.
constructor(private cdr: ChangeDetectorRef) {
this.cdr.detach();
}
To reattach the component when a change is needed, use reattach()
: